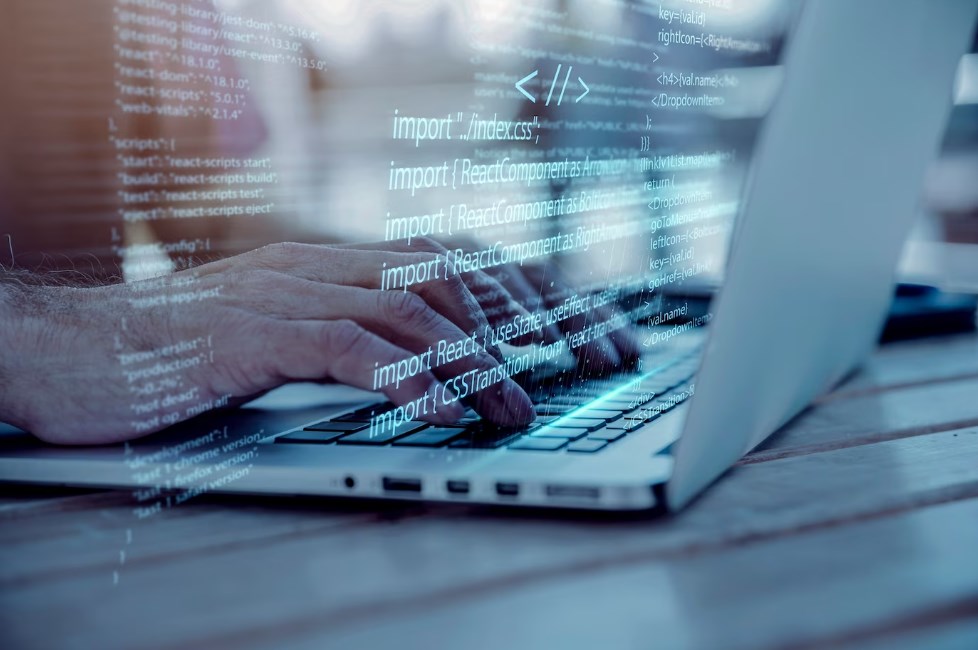
Crafting Your Own Dropdown Navigation Menus with CSS3
Designing sleek, functional dropdown navigation menus is a crucial skill for modern web developers. This guide offers a step-by-step walkthrough on creating your own dropdown menu with nothing but HTML and CSS3, eliminating the need for additional Javascript coding.
Upgraded and Animated Navigation Menu with CSS3
An enhanced and animated variant of the dropdown navigation system has recently been developed. Prioritizing this updated version may yield more interactive and visually appealing results.
Crafting a Dropdown Navigation System Without Javascript
Today’s tutorial focuses on setting up a dropdown navigation layout without incorporating any additional Javascript components. The setup is image-free and employs minimal HTML elements, making it both straightforward and efficient.
Minimalistic HTML Structure
The HTML framework employed here is minimalist, easy to comprehend, and fully functional even without styling.
<ul id="navBar">
<li><a href="#">Main</a></li>
<li>
<a href="#">Sections</a>
<ul>
<li><a href="#">Styling</a></li>
<li><a href="#">Visual Design</a></li>
<li><a href="#">Tools for Development</a></li>
<li><a href="#">Web Layout</a></li>
</ul>
</li>
<li><a href="#">Portfolio</a></li>
<li><a href="#">Bio</a></li>
<li><a href="#">Get in Touch</a></li>
</ul>
In addition, this structure is also semantic, meaning it conveys correct meanings and relationships, even in the absence of styling at this juncture.
Clean, Accessible HTML Elements
While only the “Sections” in this example include a sub-list, it’s straightforward to append sub-lists to any of the main items.
Styling Elements with CSS3
Here’s a snippet of how you can style your menu using Cascading Style Sheets:
/* Primary Styling */
#navBar {
/* properties */
}
#navBar li {
/* properties */
}
#navBar a {
/* properties */
}
/* Sub-menu Styling */
#navBar ul {
/* properties */
}
#navBar ul li {
/* properties */
}
/* Other stylings */
Note: To provide a complete guide, the comments in the CSS snippet represent where the styling properties should go.
Clearing Floated Elements
It’s crucial to clear any floated elements to maintain a structured layout. This ensures that no unintended layout issues occur, particularly in older browsers.
/* Clearing Floats */
#navBar:after {
/* properties */
}
Key Takeaways:
- Prioritize the updated and animated version of the CSS3 dropdown menu for enhanced interactivity;
- Crafting a dropdown menu requires no Javascript; CSS3 and minimal HTML suffice;
- Employ a clean, semantic HTML structure for better accessibility and SEO benefits;
- Styling is done primarily through CSS3, with properties in appropriate sections;
- Always clear floated elements to prevent layout issues, especially in older browsers.
Enhanced and Animated Dropdown Navigation Guide
While the earlier rendition of this navigation setup still holds up well, there’s a new version available that features dynamic animations. It might be worth exploring that first to see if it suits your project requirements better.
Constructing a Dropdown Navigation Using CSS3
Today’s guide aims to show you a comprehensive way to set up your own dropdown navigation system using solely CSS3—no additional Javascript or imagery is required. The HTML markup is minimalistic, making it easily understandable and editable.
Minimalistic HTML Blueprint
Upon examining the HTML blueprint, it becomes clear that there are no unnecessary elements. The design is minimalistic, facilitating a clean, straightforward experience for both developers and end-users.
<ul id="nav-list">
<li><a href="#">Home</a></li>
<li>
<a href="#">Categories</a>
<ul>
<li><a href="#">Styling Sheets</a></li>
<li><a href="#">Graphic Design</a></li>
<li><a href="#">Dev Tools</a></li>
<li><a href="#">Web Architecture</a></li>
</ul>
</li>
<li><a href="#">Work</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Contact</a></li>
</ul>
Importance of Semantic HTML
One of the notable features is the semantic integrity of the HTML structure. This ensures that even without any styling elements, the content still makes logical sense, thereby improving accessibility and SEO.
Styling With CSS3
Let’s proceed to applying styles for our navigation. This involves setting properties for the main container, list items, and hyperlinks.
/* Main Container */
#nav-list {
width: 100%;
margin: 0;
padding-top: 10px;
list-style-type: none;
background: #111;
background: linear-gradient(#444, #111);
border-radius: 50px;
box-shadow: 0 2px 1px #9c9c9c;
}
/* List Items */
#nav-list li {
display: inline;
padding-bottom: 10px;
position: relative;
}
/* Hyperlinks */
#nav-list a {
display: inline;
height: 25px;
padding-left: 25px;
padding-right: 25px;
color: #999;
text-transform: uppercase;
font: bold 12px/25px Arial, Helvetica;
text-decoration: none;
text-shadow: 0 1px 0 #000;
}
/* Hover Effects */
#nav-list li:hover > a,
*html #nav-list li a:hover { /* For IE6 */
color: #fafafa;
}
#nav-list li:hover > ul {
display: block;
}
Crafting the Dropdown Feature
To add the dropdown feature, we’ll be concentrating on creating a sub-navigation list that will be displayed upon hovering over the primary list item.
/* Sub-navigation */
#nav-list ul {
list-style-type: none;
margin: 0;
padding: 0;
display: none;
position: absolute;
top: 35px;
left: 0;
z-index: 99999;
background: #444;
background: linear-gradient(#444, #111);
border-radius: 5px;
}
#nav-list ul li {
display: block;
margin: 0;
padding: 0;
box-shadow: 0 1px 0 #111111, 0 2px 0 #777777;
}
#nav-list ul li:last-child {
box-shadow: none;
}
#nav-list ul a {
padding: 10px;
height: auto;
line-height: 1;
display: block;
white-space: nowrap;
text-transform: none;
}
/* For IE6 and IE7 */
*html #nav-list ul a,
*:first-child+html #nav-list ul a {
height: 10px;
width: 150px;
}
/* Hover Effects for Sub-navigation */
#nav-list ul a:hover {
background: #0186ba;
background: linear-gradient(#04acec, #0186ba);
}
Final Cleanup and Compatibility
Finally, to ensure that our design is compatible with various browsers, including older versions of Internet Explorer, we add some additional CSS properties. This clears floated elements and ensures the layout remains intact.
#nav-list:after {
visibility: hidden;
display: block;
font-size: 0;
content: ” “;
clear: both;
height: 0;
}
* html #nav-list,
*:first-child+html #nav-list {
zoom: 1;
}
That concludes the detailed guide on constructing a dropdown navigation using CSS3. The walkthrough has covered everything from basic HTML structure to advanced CSS styling. Now, the navigation is ready to be incorporated into your next web development project.
CSS Geometric Shapes to Enhance Navigation Interface
The triangle that appears alongside the sub-navigation is a geometric design element created using Cascading Style Sheets (CSS) version 3. This design element aims to enhance the user interface and boost usability, guiding the user’s eye to the drop-down elements.
The triangle is rendered using the ‘:after’ pseudo-element, in which specific attributes are set to zero and border styles are manipulated to create the triangle shape.
#navigationList ul li:first-child a:after {
content: “”;
position: absolute;
left: 30px;
top: -8px;
width: 0;
height: 0;
border-left: 5px solid transparent;
border-right: 5px solid transparent;
border-bottom: 8px solid #444;
}
The color of the triangle changes upon hovering over it, providing a visual cue to the user that it’s an interactive element:
#navigationList ul li:first-child a:hover:after {
border-bottom-color: #04acec;
}
Ensuring Accessibility for Legacy Browsers Like IE6
IE6 presents a particular challenge when it comes to hover effects on list items (li elements) since it doesn’t natively support this feature. Although the primary focus of this guide is to build a dropdown navigation without the use of JavaScript, an exception is made here to ensure compatibility with older versions of Internet Explorer.
Here’s the scripting snippet that addresses this issue. It relies on jQuery, a widely-used JavaScript library:
<script type=”text/javascript” src=”http://code.jquery.com/jquery-latest.min.js”></script>
<script type=”text/javascript”>
$(document).ready(function() {
if ($.browser.msie && $.browser.version.substring(0, 1) < 7) {
$(‘li’).has(‘ul’).on(‘mouseenter’, function() {
$(this).children(‘ul’).show();
}).on(‘mouseleave’, function() {
$(this).children(‘ul’).hide();
});
}
});
</script>
While this script is primarily for IE6, it’s worth mentioning that IE6 usage is dwindling, and you might consider skipping this step.
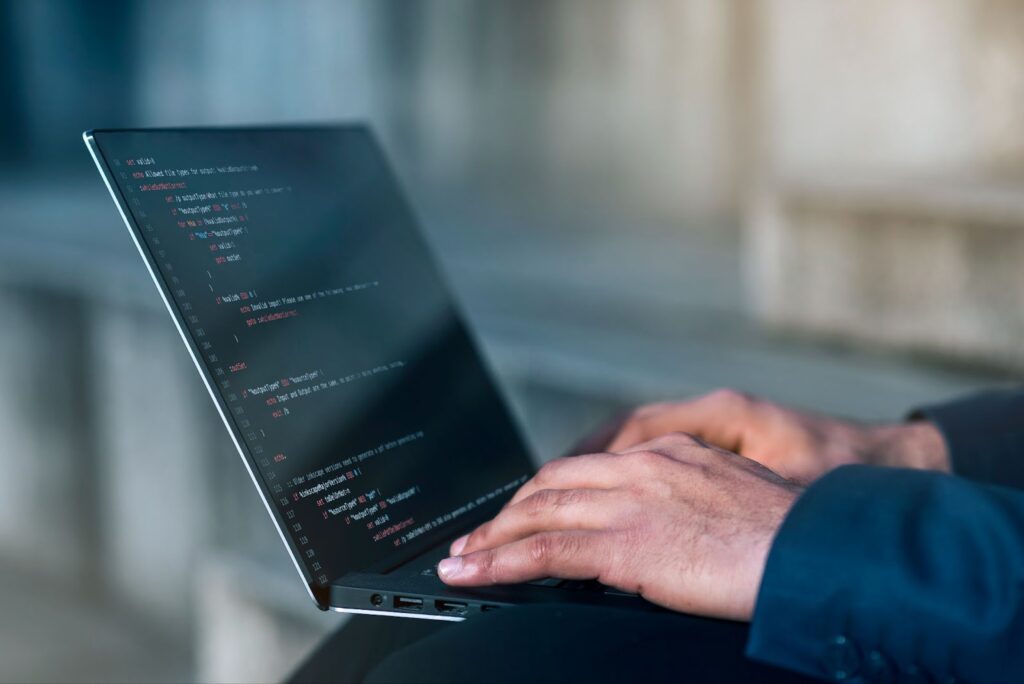
Considerations for Targeting IE6 and IE7 Specifically
Apart from the jQuery workaround, additional styling rules have been incorporated to target IE6 and IE7 explicitly. These rules employ the zoom property, ensuring that the layout is not broken in these older versions:
* html #navigationList { zoom: 1; } /* Targeting IE6 */
*:first-child+html #navigationList { zoom: 1; } /* Targeting IE7 */
For those concerned about stylesheet validation, other techniques like conditional comments can be employed to maintain a valid stylesheet while targeting these legacy browsers.
Conclusion
This tutorial aims to offer an in-depth guide to creating a feature-rich and accessible dropdown navigation, complete with a focus on browser compatibility and modern styling techniques. For additional queries or feedback, the comments section is open. Thank you for engaging with this content.