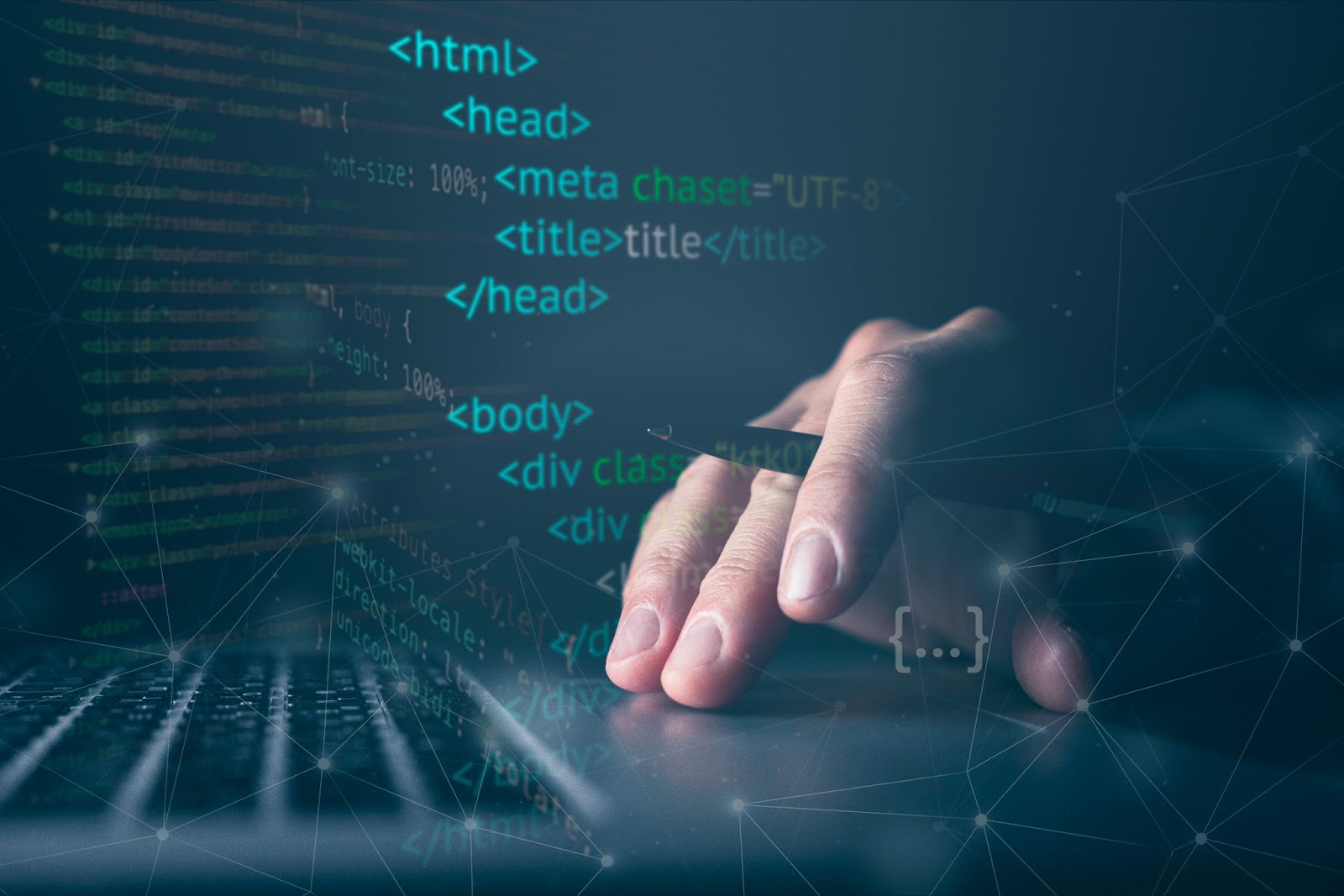
Unlocking the Potential of Interactive Image Map Hints
Tooltips wield significant influence in enhancing your web designs, a fact that’s hardly novel. Employ them judiciously, and you’ll witness a notable enhancement in user experience.
We’ve previously explored the art of crafting aesthetically pleasing CSS3 tooltips. Today, you’ll delve into the realm of creating an image map replete with pins and accompanying tooltips.
Concept Overview
In recent times, there has been a growing interest in image maps featuring tooltips, driven by some notable examples on renowned platforms such as the Firefox website. This inspiration led to the development of a unique and intuitive way to create these tooltips. The primary objective behind this initiative is to offer a solution that’s not just user-friendly, but also doesn’t demand any technical expertise for updates.
For users who are not well-versed in coding or development, this approach provides a sigh of relief. The process doesn’t require one to possess any development skills. Instead, all that’s required is the addition of a specific division (div) with the desired content. Furthermore, setting two specific HTML5 attributes will finalize its positioning. Essentially, it offers a seamless experience even for those who are novices in the web development realm.
Dive into HTML5 Data Attributes and jQuery
HTML5 introduces an exciting feature termed “custom data attributes.” This unique feature offers a way to embed and store arbitrary metadata, ensuring your Javascript code remains clean and easy to understand. This development means that there’s no longer a need to rely on attributes like “rel” or “title” exclusively for Javascript integrations.
To give a clearer perspective, here’s a breakdown of its syntax:
<div data-example="value"></div>
To retrieve the embedded value using jQuery, one would employ the following method:
var extractedValue = $('div').data('example');
For the purpose of this discourse, the focus will be on utilizing these custom data attributes to embed essential data such as the positioning coordinates for the pins on our map. This approach not only simplifies the coding process but also ensures that the user experience remains smooth and intuitive.
A Deep Dive into Interactive Map Styling
Maps are integral to many websites, providing valuable context, directions, or information on various geographical entities. Let’s dissect and enhance an example of an interactive map.
HTML Structure:
<div id="wrapper">
<img width="920" height="450" src="world-map.jpg" alt="World continents">
<div class="pin pin-down" data-xpos="450" data-ypos="110">
<h2>Europe</h2>
<ul>
<li><b>Area (km²):</b> 10,180,000</li>
<li><b>Population:</b> 731,000,000 </li>
</ul>
</div>
</div>
Breakdown:
- #wrapper: This serves as the container holding the map and all associated elements. It uses relative positioning, which ensures that child elements positioned absolutely are relative to this wrapper;
- img: Represents the actual map image which sets the scene. It acts like the background upon which pins and tooltips will be overlaid;
- .pin: A crucial element that denotes a specific location on the map. Nested within are details about that location. The ‘pin-down’ class indicates the visual type or style of the pin;
- data-xpos and data-ypos: HTML5 custom attributes that facilitate precise pin positioning. Here, the pin is 450px from the left and 110px from the top.
CSS Styling:
#wrapper {
position: relative;
margin: 50px auto 20px auto;
border: 1px solid #fafafa;
box-shadow: 0 3px 3px rgba(0,0,0,.5);
}
.pin {
display: none;
}
.tooltip-up, .tooltip-down {
position: absolute;
background: url(arrow-up-down.png);
width: 36px;
height: 52px;
}
.tooltip-down {
background-position: 0 -52px;
}
.tooltip {
display: none;
width: 200px;
cursor: help;
text-shadow: 0 1px 0 #fff;
position: absolute;
top: 10px;
left: 50%;
z-index: 999;
margin-left: -115px;
padding: 15px;
color: #222;
border-radius: 5px;
box-shadow: 0 3px 0 rgba(0,0,0,.7);
background: #fff1d3;
background: linear-gradient(top, #fff1d3, #ffdb90);
}
.tooltip::after {
content: '';
position: absolute;
top: -10px;
left: 50%;
margin-left: -10px;
border-bottom: 10px solid #fff1d3;
border-left: 10px solid transparent;
border-right: 10px solid transparent;
}
.tooltip-down .tooltip {
bottom: 12px;
top: auto;
}
.tooltip-down .tooltip::after {
bottom: -10px;
top: auto;
border-bottom: 0;
border-top: 10px solid #ffdb90;
}
.tooltip h2 {
font: bold 1.3em 'Trebuchet MS', Tahoma, Arial;
margin: 0 0 10px;
}
.tooltip ul {
margin: 0;
padding: 0;
list-style: none;
}
Styling Insights:
- #wrapper: The container is styled with a gentle shadow for depth and a light border. The automatic margins center it horizontally;
- .pin: Initially hidden, the pins are made visible during interactions like mouse hovering;
- .tooltip-up & .tooltip-down: Absolute positioning allows these tooltip arrows to hover over the map. The image used here contains both up and down arrows. The .tooltip-down style shifts the background to show the downward arrow;
- .tooltip: A hidden informative box providing details about the selected location. Styled with a gradient background, shadow for depth, and a centrally positioned triangular indicator. The latter’s direction changes based on the tooltip’s position (up or down).
In sum, this code provides a rich, interactive map with specific location pins. The tooltips offer valuable insights upon interaction, making geographical data both visually appealing and informative.
jQuery Code Overview and Implementation
Introduction to the jQuery Code:
The provided jQuery code serves as an elegant method to add interactive pins with tooltips on an image. This functionality enriches user experience by allowing annotations on the image that can display additional information when hovered over.
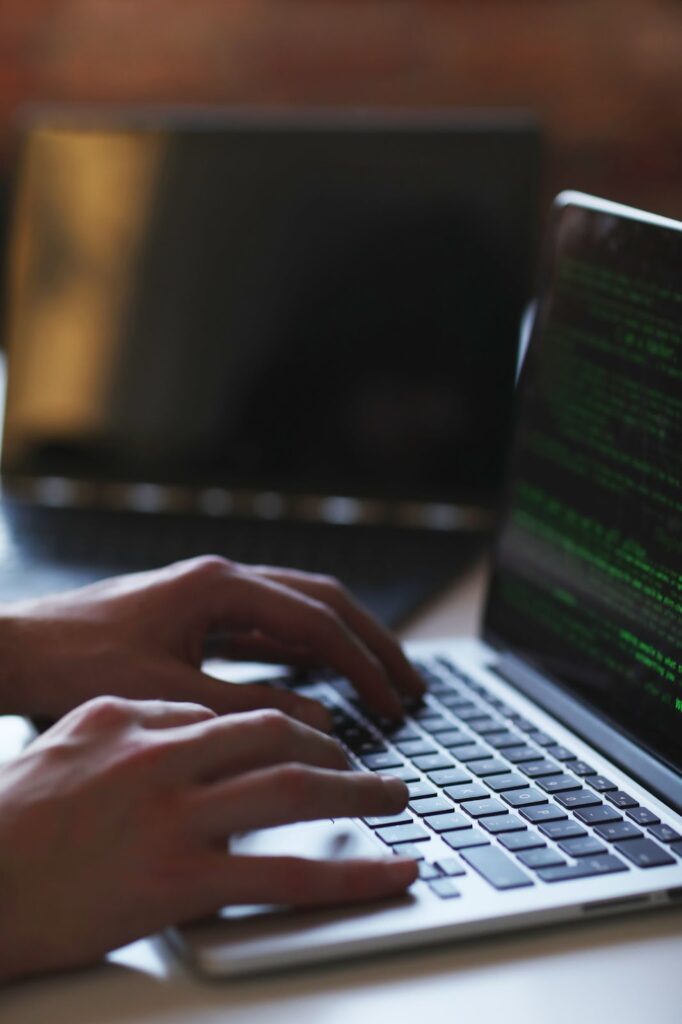
jQuery Code Breakdown:
When the webpage loads, the following actions take place:
Dimension Setting: The dimensions (width and height) of the #wrapper element are set to be the same as the image inside it. This ensures that the wrapper completely covers the image, serving as a foundational layer for the subsequent pins and tooltips.
$('#wrapper').css({
'width': $('#wrapper img').width(),
'height': $('#wrapper img').height()
});
Tooltip Direction Determination: The code then determines the direction of the tooltip (either ‘up’ or ‘down’) based on the class of the .pin elements.
var tooltipDirection;
for (i = 0; i < $(".pin").length; i++) {
if ($(".pin").eq(i).hasClass('pin-down')) {
tooltipDirection = 'tooltip-down';
} else {
tooltipDirection = 'tooltip-up';
}
}
Tooltip Appending: Based on the direction and the HTML5 data attributes data-xpos and data-ypos (which dictate the positioning of the pins), the tooltips are appended to the #wrapper.
$("#wrapper").append( ... );
Tooltip Interaction: The tooltips exhibit a hover effect. When a user hovers over a tooltip (whether it’s facing ‘up’ or ‘down’), the tooltip content fades in. When the cursor moves away, the tooltip content fades out.
$('.tooltip-up, .tooltip-down').mouseenter( ... ).mouseleave( ... );
Usage and Implementation Guide:
- Browser Compatibility: This jQuery method boasts impressive compatibility, functioning seamlessly even on older browsers such as IE6. However, it’s worth noting that certain CSS3 attributes, such as gradients and shadows, may not render on these outdated platforms;
- Image Setting: Within the <div id=”wrapper”> element, insert the desired image to be annotated with pins;
- Pin Content Addition: To introduce a pin with a corresponding tooltip, encapsulate the tooltip’s content inside a <div class=”pin” data-xpos=”X-COORDINATE” data-ypos=”Y-COORDINATE”> tag. The data-xpos and data-ypos attributes are responsible for dictating the tooltip’s position relative to the image.
jQuery Automation: With the above elements in place, the jQuery code works its magic:
- The wrapper acquires the dimensions of the contained image;
- Original content inside the .pin class gets concealed via CSS, and new elements based on this content are dynamically generated;
- The dynamically generated pins and tooltips are placed on the image using the HTML5 data attribute coordinates;
- Tooltips seamlessly appear and disappear in response to user hover actions, courtesy of the mouseenter and mouseleave events.
Conclusion
I trust that you found this tutorial enjoyable, and please don’t hesitate to share your thoughts or suggestions in the comments section. Your engagement and feedback are greatly appreciated. Thank you for taking the time to read!